Developers follow different approaches to software development. Some stick to established philosophies, while others come up with their own.
My philosophical influences come from Lean Domain-Driven Design (DDD), Test-Driven Development (TDD), The Agile Software Development Life Cycle (SDLC), Developer Operations (DevOps), and more.
Here’s my software development philosophy. What’s yours?
People First
I create software that benefits others and strives for a positive work environment. Your needs come first.
Invest Lightly
Technology changes constantly; I limit my keystrokes and produce thoughtful low-maintenance software architectures.
Stay Liquid
I avoid technical debt like COVID. It’s frighteningly common to witness multi-million-dollar software projects scrapped and rebuilt for millions again.
A mountain of technical debt is no laughing matter; it threatens the longevity of your business. I recommend paying your technical debt now. Rewriting software is often more expensive than paying technical debt.
Everything Changes
When building code, I assume change is coming sooner than expected. I craft flexible solutions that economically fulfill new business requirements.
Simple Security
Simple security is often an oxymoron, but there are solutions.
I create layers to protect data for access rule clarity rather than using software configuration files.
I require clients to identify themselves before calling my services. Legacy deprecation is hard enough without introducing a game of whack-a-mole or resorting to scream tests during migration activities.
I often remind myself that the only secure computer is a dead computer, and you better be sure it’s demolished, in the ocean, encased in cement, and buried.
Use the Right Tool
Languages are programming tools that help us craft solutions. I consider the appropriate tool for a job and am acutely aware of The Law of Instrument.
In software development, many people use one tool and master it. Specialization is lucrative; some developers will profit from this approach. However, I prefer to follow the 80-20 rule. I learn several tools to unlock full-stack development proficiency.
I’m pragmatic, yet I apply principles contextually.
The Mythical Man-Month
Many believe adding more people will speed up a project. I don’t blame them; it seems like the logical outcome; however, it’s never true on a short timeline.
I’m acutely aware of Brook’s Law. Adding new people to a team will initially slow it down.
I simplify onboarding activities to lower the impact of adding new team members.
Cut With The Grain
I build solutions with an eye for opportunity. If hosting software is cheap with the cloud, do it; otherwise, seek other less expensive solutions.
I skip dogma when allocating resources and address inefficient resource allocations.
Measure Then Commit
When building software, I focus on critical decisions, then fire tracer bullets to determine options.
I pass proposals by my team to eliminate blind spots.
I measure twice and then commit.
All The Logs In The Forest
I collect all log sources as I never know what they might reveal when debugging a problem.
While information is essential, too much information drives me crazy. I appropriately classify logs with DEBUG, INFO, WARN, ERROR, and FATAL.
I implement general-purpose logging with aspect-oriented programming and control planes, keeping most logging concerns out of applications.
Start Fresh
When I dust off a software development tool, I approach it as a newbie. I search for a modern tool and remind myself that my tools may soon be obsolete.
I embrace a beginner’s mind and avoid the sunk cost fallacy.
I use principles to guide me rather than knowledge of a tool.
Solutions Looking For a Problem
I ask myself a few simple questions before building anything.
- Is this feature valuable?
- Does this feature solve a significant user or business problem?
- Does this feature increase or preserve revenue?
If a feature isn’t valuable, I postpone its development.
Reframe The Problem
I can’t eat a garden with one bite.
When I solve a complex problem, I break it into bite-sized bits. I work methodically to create discrete solutions to simple problems.
I reframe complex problems into economical and educational solutions. Later, I apply bronze, silver, or gold-plated solutions depending on failure impacts.
Use Cases
It’s vital to limit software use cases. A command-line utility’s use cases are easy to restrict, while user interfaces are not. I limit use cases to reduce bugs.
A clear definition of use cases defines a contract between myself and users. I document a use case when people use my software in novel ways.
I create extendable features when developing general-purpose software.
Constraints Are Good
If it’s flapping around, I nail it down. I identify and document constraints before writing any code. Defining constraints simplifies and improves decision making.
I obsess over resource constraints and costs. Before deploying a software architecture, I review technical limitations and business rules to discover absolute blockers or quality impediments.
I regularly validate previously defined constraints. If something changes in my favor, I’ll forge an optimized path.
Versioning Most Things
I version most things. I version software, hardware, and workflows.
I pin package versions and apply the principle: if it’s not broken, don’t fix it.
I update insecure packages proactively.
I like comver.
Name Things With Purpose
When naming variables, I name them in ways that make them easy to rename. I call things exactly and avoid overloading or overusing the same variable name.
Fire Tracer Bullets
I use tracer bullets to punch a hole in the walls between myself and a solution. I use scattershot shotgun methods to prove use cases, theories, and the viability of a solution before investing time and money in building robust solutions. I pick a requirement far from the final solution and meet it head-on.
There Are No Big Problems
As Henry Ford once said, there are just a lot of minor problems. Breaking down what looks like big problems into manageable problems makes software developers look like wizards. 🧙♂️
If I face a complex problem, I solve a minor problem and work from there.
I like to say, “You can’t eat a garden with one bite!”
Drive Human Value
I prioritize end-user needs and simplicity over complexity. I consider the human-centric value of features before building anything.
Single Responsibility
I isolate software components with a single responsibility and create single-responsibility user interfaces to reduce complexity. I divide tools logically for users and software developers and follow SOLID principles when creating object-oriented software solutions. Many software problems are caused by failing to follow these principles.
Take Responsibility
Development Operations (DevOps) has transformed software development. Modern software development practices have greatly benefited from DevOps.
DevOps requires software development teams to take full responsibility for their creations. The DevOps maturity model increases the quality of products and increases the speed of delivery to the market.
I guide team members towards continuous improvement of build pipelines, testing methodologies, and other DevOps best practices.
There’s Always A Design
Designing a maintainable, malleable, and easy-to-understand system is of paramount importance. A design always exists. An unplanned design is terrible, but it is still a design. A poor design becomes a massive liability for a company and will slow a development team to a crawl.
I insist on a good design upfront. My primary goals include making a system sufficiently flexible, understandable, and affordable.
Be Empathetic
Empathy for people is a cornerstone of a robust software development philosophy.
I work diligently to understand what people need. I ask why five times to understand the real problems while keeping the big picture in mind.
I write code that anyone can understand by eliminating questions collected through solicited feedback.
Step Slowly
When solving complex problems, I step slowly through code. I make manageable changes. Slowly stepping through code helps isolate errors and correct them individually.
I don’t cut corners when a path is clear. Quality is more important than speed; speed emerges through grade.
Slow is smooth, smooth is fast. — Bob Martin
Automate Almost Everything
I automate tasks religiously. I consider a different approach if it’s expensive to automate a solution.
Test For Life
I like focusing on solving problems. Without robust integration tests, unit tests, and other tests, we spend time creating problems for ourselves.
I write tests because manually running an app after every change is tedious.
An automated test suite helps me sleep at night. Time spent coding without testing increases the time spent working on bugs, investigating production issues, and troubleshooting broken builds. I prevent future pain by testing; therefore, I test for life.
Exceptions Are The Exception
I write code to avoid mistakes. Instead of throwing exceptions in the name of defensive programming, I change software designs to prevent thrown exceptions. After all, users depend on software to work and simplify their life; let’s keep it simple.
However, I bubble up exceptions with maximum traceability when required.
I implement messaging patterns, isolate control of resources, and identify other creative ways to avoid errors before throwing exceptions.
Decouple Everything
I refactor tightly coupled code to reduce complexity and prepare it for change. When tight couplings exist for no good reason, I decouple them.
I summarily dismiss software libraries that aren’t dependency injection-ready.
I ask myself five times why I’m coupling code before committing to tight coupling.
No Perfect Solutions
Picking the best solution, at the moment, with all the facts laid out, is the way to avoid analysis paralysis.
I don’t believe in the Nirvana fallacy and make the best decision possible at The Last Responsible Moment, yet at the same time, I take big architectural choices very seriously.
Solutions can’t be fast, cheap, and good simultaneously, yet I strive for the right balance based on functional requirements.
Conway’s Law Is True
Conway’s Law states that the organization’s communication structure influences a system design.
Any organization that designs a system (defined broadly) will produce a design whose structure is a copy of the organization’s communication structure.
— Melvin E. Conway
If communication flows are complex, so are systems.
I inspect organizational information flow to understand its procedures. If the flow could be more efficient, I propose a better solution.
Open Source is Life
I make use of Opens Source software whenever possible. I ensure the Open Source software I use is well maintained and make safe bets on a project’s longevity. I’ll contribute to a project if it aligns with my development needs and enjoy the synergies that Open Source software development models provide to businesses and developers.
I promote InnerSource first culture that leads to Open Source contributions.
Can != Should
The software development world has many tools, methods, design patterns, and best practices. Frequently choice overload leads software developers to believe one tool can rule them all. 💍
Picking the wrong tools to solve your problem leads to technical debt and wasted time. Before tackling a problem, I will research the standard methods and choose the best tools for a specific situation.
Confusion Is Painful
Software development is overly complicated as-is. I follow the principle of least astonishment.
If I forge an unconventional path, I prepare a steelman argument, keep it simple, and document why.
Code is language. The code I write speaks succinctly to people first then a compiler.
I keep my variables consistent, methods clear, and configuration files clean, and I make sound choices to eliminate inconsistencies.
Inconsistency is an enemy of excellent software design.
Pair Programming FTW
I enjoy a productive pair programming session. I love sharing my hard-earned wisdom. Programming in the modern age is a self-induced chaos test meant to push my ability to understand EVERYTHING to the limit. When I help someone avoid the pain I’ve experienced, it brings me joy.
Another pair of eyes will make code easier to maintain and lower the total cost of ownership. Pair programming, when done right, will level up your team while turning it into a high-performing powerhouse.
A Software Development Philosophy Evolves
My software development philosophy is evergreen. It changes after learning new things. This list contains a small fraction of my overall development philosophy. As always, a great magician only reveals some of their tricks.
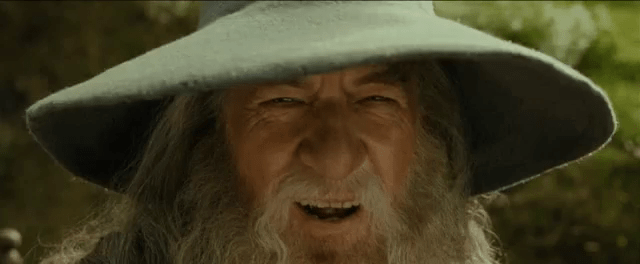
I refine my software development philosophy regularly in the spirit of continuous improvement.
Develop your philosophy
Here are a few books to help you develop your software development philosophy.
- The Pragmatic Programmer
- Clean Code
- Clean Architecture
- Solution Architect’s Handbook
- The Elements of Programming Style
Related Content
- List of software development philosophies - Wikipedia
- A Philosophy of Software Design | John Ousterhout | Talks at Google
- Product
- Software
- SOLID - Wikipedia
- Summary of ‘Clean code’ by Robert C. Martin - gists · GitHub
- Principles of Security - Cornell Computer Science
- Seven basic principles of software engineering
- 10 Crucial Software Development Principles
- Software Architecture Principles That Every Programmer Should Follow
- Software engineering principles to live by
- The 10 Principles of Observability
- Principles behind the Agile Manifesto
- Software Architecture
- Graphics Design
- Operations
- Soft Skills